Data structures are one of the most important and fundamental concepts in programming. The data structures are used to keep the data in the application.
Data management is an important task for all software systems and applications in the design and development phase. We can find the application of the data structure almost everywhere we could imagine. For example, if we are building a student management system and want to store the data in the application, we will use data structures for this purpose.
Data structures help us to organize our data efficiently and in a better way. The use of data structures makes our program faster and efficient. Python is the most efficient programming language when it comes to data structures.
Python supports the fundamental data structures more efficiently and easily as compared to the other programming languages. We would learn to use data structure through simple examples.
If you want to know more about data structures in general and how they work in concept, check out my article on 8 essential data structures.
Data Structures in Python
There are multiple types of data structures that are used in programming. Some of the data structures are linear and some are non-linear.
The linear data structures follow the sequence and sequentially store the data whereas, the non-linear data structures do not follow any structure. The common example of linear data structure is an array, linked list, and stack.
The most well-known and common non-linear data structures are graphs, trees, and heap. The arrays, linked list, queues, stack, graphs, and tress are the most common and widely used data structures.
Python also has some specific data structures i.e. list, tuple, and dictionary.
The arrays, list, hash table, linked list, and queues are the most commonly used data structures in Python. Let’s discuss these data structures in Python respectively.
The Array Data Structure
An array is used to store the data sequentially. Almost every programming language offers the luxury to use arrays. An array stores a similar type of data in a single container and later on the data is accessed by the index number. An index is primarily the location of the stored item in an array. We can think of an array as a container that has multiple boxes placed in a sequence. Each box stores a particular value. The number of the particular box can be identified by a sequence. The box that is placed first is at the first number and so on. The arrays in Python are braced by the array module. A similar type of data can be kept in an array. The data could not be of heterogeneous type.
When it comes to creating or defining an array, it means that we also want to define an appropriate data type for it.
Let’s create a student array to store the marks of a student. The array index value starts from 0.
import array as arr
# creating a float type array
marks = arr.array(‘d’, [90,91,92,45,87,89.5,25,20.5])
# printing the array
print ("The marks of the student are : ", end =" ")
#using for loop to access the array indexes
for i in range (0, 8):
#accessing the data stored at every index by using iterator i
print (marks[i], end =" ")
Output
The output displays the data stored in the array. The arguments of the range function are 0 and 8. It means that the data from the array should be fetched from index 0 to 8.
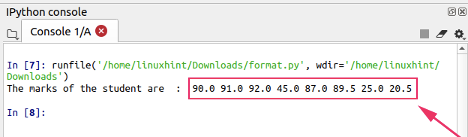
import array as arr
# creating an array of integer type
num = arr.array('i', [1,2,3,4,5,6,7,8,9])
# printing the array
print ("The data inside the array is : ", end =" ")
#using for loop to access the array indexes
for i in range (0, 8):
#accessing the data stored at every index by using iterator i
print (num[i], end =" ")
Output
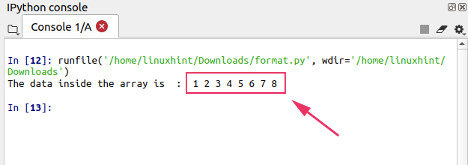
We can also access the particular of an array by using the []. It will return us only a single item from the specified index.
import array as arr
# creating an array of integer type
num = arr.array('i', [1,2,3,4,5,6,7,8,9])
# accessing the first element of array
print(num[0])
# accessing the second element of array
print(num[1])
# accessing the third element of array
print(num[2])
# accessing the fourth element of array
print(num[3])
# accessing the fifth element of array
print(num[4])
# accessing the sixth element of array
print(num[5])
# accessing the seventh element of array
print(num[6])
Output
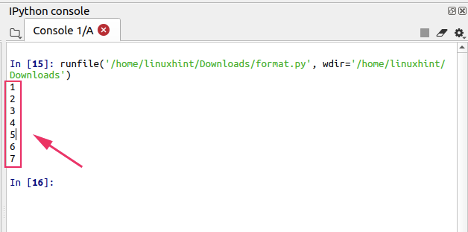
Lists
The list is one of the fundamental and most used data structures in Python. List stores the data in a sequential way and it can contain the multiple types (integer, float, string) data.
We can formally create a list in python by using “[]” known as square brackets. The fundamental difference between the list and array is that the array is static whereas, a list is a type of dynamic data structure.
Once an array is declared and initialized then we cannot add the data into an array at run time. On the other hand in the list, the data could be added dynamically. We can easily access any index of the list.
Let’s create a Python list.
#creating a list
students=["John","Mark","Taylor","Smith"]
#prinitng the list
print(students)
#accessing the specific index of list
print(students[2])
Output
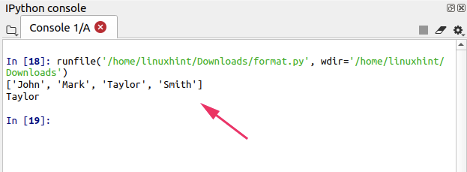
Let’s declare a list of multiple types and insert some data into the list after declaring and initializing the list. The append() function puts the data at the end of the file where it doesn’t remove the already present data. The insert() function puts data at the specific index given by the programmer.
#creating a list
students=[1,"John",2.5,"Mark",3,"Taylor",4,"Smith"]
#appending the list
students.append(8)
#inserting the number 9 at position 1
students.insert(1,9)
#prinitng the list
print(students)
Output
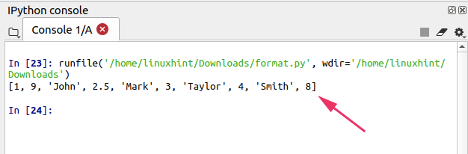
The hash table
The hash table is one of the important types of data structures. It makes our search of the data faster. The hash table contains the data in a specific form of key-value pairs. The key is the index value. The index value for each type of data is different. Therefore, searching the data based on a unique index value is faster and easy. Similarly, the insertion and deletion of the data in the hash table is also a faster operation, because the index value becomes the key.
In Python, the implementation of the hash table is very easy and straightforward. The hash table is implemented using the dictionary data types in Python. The dictionary data type store the data in the forms of key-value pairs. While creating a data dictionary, first we create the key and then define the value. Let’s create a hash table in Python using the data dictionary data type. We are creating a data dictionary of a student to store student’s information. In the given example, the name, age, class, and semester are the key or index values.
#declaring a hash table or data dictionary for student
student_dict={'name':'kamran','age':25,'class':'MS','smeseter':'Fourth'}
#the data is accessed by using the key
#printing the name of student
print(student_dict['name'])
#printing the age of student
print(student_dict['age'])
#printing the class of student
print(student_dict['class'])
#printing the smeseter of student
print(student_dict['smeseter'])
Output
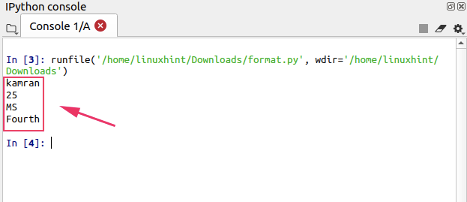
The value can be accessed easily by using the key. The key is specified in pair of brackets[] and it returns the value.
We can easily update the dictionary by adding a key value pair. Let’s add the student email.
#declaring a hash table or data dictionary for student
student_dict={'name':'kamran','age':25,'class':'MS','smeseter':'Fourth'}
#the data is accessed by using the key
#printing the name of student
print(student_dict['name'])
#printing the age of student
print(student_dict['age'])
#printing the class of student
print(student_dict['class'])
#printing the smeseter of student
print(student_dict['smeseter'])
#updating the dictionary
#adding new key value pair for email
student_dict['email'] = 'kamran@xyz.com'
#printing the student email
print(student_dict['email'])
Output

Likewise, we can delete the data from the dictionary by using the del keyword. Let’s delete the age key-value pair. #declaring a hash table or data dictionary for student
#declaring a hash table or data dictionary for student
student_dict={'name':'kamran','age':25,'class':'MS','smeseter':'Fourth'}
#the data is accessed by using the key
#printing the name of student
print(student_dict['name'])
#printing the age of student
print(student_dict['age'])
#printing the class of student
print(student_dict['class'])
#printing the smeseter of student
print(student_dict['smeseter'])
#updating the dictionary
#adding new key value pair for email
student_dict['email'] = 'kamran@xyz.com'
#printing the student email
print(student_dict['email'])
#deleting age value
del student_dict['age']
#printing the whole dictionary
print(student_dict)
Output
In the output it can be observed that the age key-value pair is deleted.
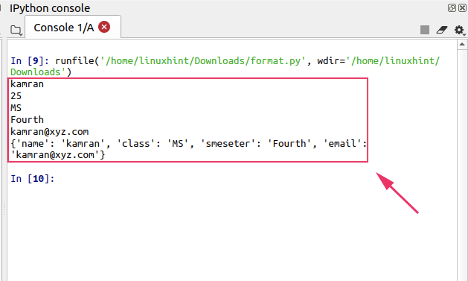
Queues in Python
A queue is a type of data structure. It is a form of a linear data structure. The queue performs its task based on the principle of First in, First out also known as FIFO. In the queue, the data items are stored in a sequence.
The data that has been put first, goes out first. We can find the application of queue in our daily life. Imagine the queue of the customers outside any bank or any market. The customer that reaches out first, will be given services first. The customer who comes last, occupy the space at last of the queue.
We could use a queue to perform the following operations:
- Enqueue: The enqueue operation adds the element in the queue.
- Dequeue: The dequeue elements removes the item from the queue.
The queues in Python are implemented using the list data type. The list data structure has been discussed in detail above. Now let’s form the queue using the list. Let’s write a simple Python program to implement queue by using list data structure. The append() function is used to enqueue the data in the list whereas, the pop() function performs the dequeue operation. The elements would be eliminated in the same order as they were put in.
#declaring the queue
que = []
#adding the data in queue.
que.append(1)
que.append(2)
que.append(3)
que.append(4)
#printing the elements of
print(que)
#removing the elements from que
que.pop()
que.pop()
que.pop()
#printing the queue
print(que)
Output
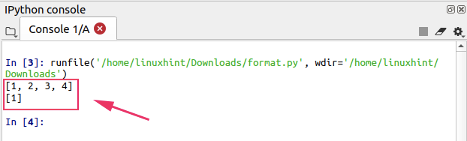
Linked List
A linked list is a type of data structure that contains the data elements in a sequence. The linked list is a dynamic data structure. Unlike the arrays, we do not need to specify the size of the linked list at the time of creation.
The data in the linked list is stored in the sequence where one data element has a connection with other data element. The linked list is implemented using the node class. Python does not provide the built-in data type or mechanism for implementing the linked list. We create the Node class and then we implement the linked list in Python. A node contains the data and the pointer to the next node.
The first node is called the head, and the last node does not contain the reference or pointer of any other node.
The node is created in a separate class and accessed in another class, which is often known as linked list class. Let’s write down a simple program to understand the usage of linked list.
#create a node class
class Node:
# initializing the node object with data and the next node
def __init__(self, data):
self.data = data
self.next = None
# creating the linked list class
class Linked_List:
# Initilizing the fisrt node (head)
def __init__(self):
self.head_node = None
# printing the linked list
def print_Linked_List(self):
temp_node = self.head
while (temp_node):
print (temp_node.data)
temp_node = temp_node.next
# declaring the mnain function
if __name__=='__main__':
# Start with the empty list
linkedlist = Linked_List()
#declaring the first node as a head node
linkedlist.head = Node(1)
#creating another node
second_node = Node(2)
#creating the reference of second node with the head
linkedlist.head.nex=second_node
linkedlist.print_Linked_List()
Output
The linked list is created successfully. The output displays the value of the node.
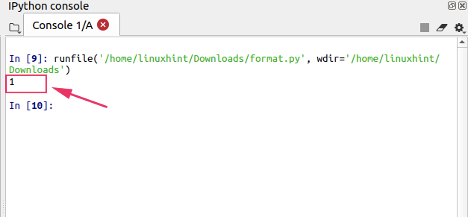
Conclusion
Data structures are the fundamentals of computer science and programming languages. Data structures are an efficient mechanism for storing and organizing data. Python supports all types of data structures. However, it has some specified data structures like lists, dictionaries, and tuples. These specified data structures are used for various purposes i.e. for organizing the data and keeping the data. This article explains the data structures in Python with examples.
This article was written by Juan Cruz Martinez (twitter: @bajcmartinez), founder and publisher of Live Code Stream, entrepreneur, developer, author, speaker, and doer of things.