Table of Contents
- Introduction to Python
- Installing Python on different Operating System
- Writing the first program in Python
- How to write comments
- Variables
- Tokens
- Conditional Statements
- Data types in Python
- Strings
- Lists
- Tuples
- Sets
- Dictionaries
- Typecasting
- Receiving Input
- Looping Statements
- Functions on Python
- Lambda Functions
- Classes and Objects
- Inheritance
- Abstraction
- Modules
- Regular Expressions
- File Handling
- Exceptions
- Conclusion
Introduction to Python
- Python is the most popular programming language for any beginner. It is easy to read and understand by any non-technical person.
- It was created in 1990 by a Dutch programmer named Guido Van Rossum.
Python is an interpreted, high-level, dynamically typed, and general-purpose programming language.
Python is a platform-independent programming language. It means it can run easily on several platforms like Windows, Linux, Mac, and many more.
Features of Python
- General Purpose: This language is used in various applications domains without being restricted to a particular domain.
- Interpreted: It means it executes instructions directly, without previously compiling a program into machine-language instructions.
- High Level: It uses natural language elements and automates crucial areas of computing such as memory allocation.
- Dynamically Typed: Python is dynamically typed which means it identifies the type of the variable based on what kind of data you have allocated to the variable.
- Object-oriented programming: Python supports OOP’s concept which means the concepts on objects, classes, and encapsulation help programs to run efficiently.
- GUI Supported: It gives a visual look to our code which makes it more convenient and easy to understand by a normal human being
Applications of Python
- Machine learning: Python can also be used to create various machine learning models and make predictions. There are libraries like Sklearn that will help us to do this task.
- Web Applications: It is used to create various levels of web applications using web frameworks like Django and Flask. It is suitable for both backends as well as frontend development.
- Automation: It can also be used to automate tasks like sending emails, filling forms, and scheduling your post on social media.
- Game development: Python is also very popular in game development. There is a module called Pygame that helps to make amazing games.
- Data Visualization: Python can also be used to visualize data. There are various libraries to do this like matplotlib, seaborn, and plotly.
Installing Python on different Operating System
Installing Python 3 on Windows
Step 1- Go to https://www.python.org/downloads/.
Step 2- Download the python version 3.6 or more.
Step 3- After the download is completed, double-click on the installer and then on the next screen check the box indicating to “Add Python 3.x to PATH” and then click on “Install Now”.
Step 4- After installation is complete, close this.
Step 6- Go to the command prompt and type “python — version ” in it. And you will see your python version it means you have successfully installed python.
Installing Python 3 on Mac
Step 1- First install brew. Go to https://brew.sh/ and follow the instructions on the first page to install it.
Step 2- Install python3 with brew
brew install python3
With this command, you have successfully installed Python on your system.
Installing Python 3 on Linux
Installing python3 using apt on the terminal.
sudo apt install python3
Writing the first program in Python
Let’s write the first “hello world” program in Python
print("hello world")
Comments can be used to make the code more descriptive and understand by a newbie programmer. So whenever any new tech guy comes and reads the code he will understand it clearly.
There are two types of comment
1- Single line comment: It is denoted by #.
# This is a single line comment
2- Multi-line comments: It is denoted by “””.
"""
This is a comment
written in
more than just one line
"""
Variables
Variables are the placeholder that is used to represent data. To assign values to a variable we use assignment operator.
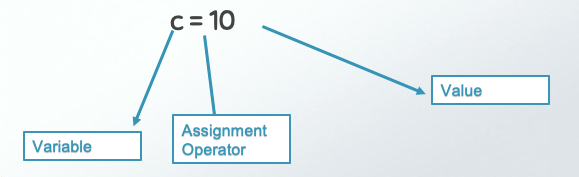
Rules for defining a variable
- When we define a variable name it must start with a letter or the underscore character
a=5 or _a=34
2. A variable name cannot start with a number, if we start with numbers then a string of digits would be a valid identifier as well as a valid number.
3. We can use alpha-numeric characters and underscores to define a variable.
4. It is case-sensitive (name and Name are two different variables).
name="Harry"
Name="Ralph"
Tokens in Python
Tokens are considered as small units of the programming language. It consists of mainly 4 parts which are Keywords, Identifiers, Literals, Operators.
Keywords
Keywords are special reserved words that have their predefined meanings. We cannot use it to define variables names.
Some of the keywords that are given below
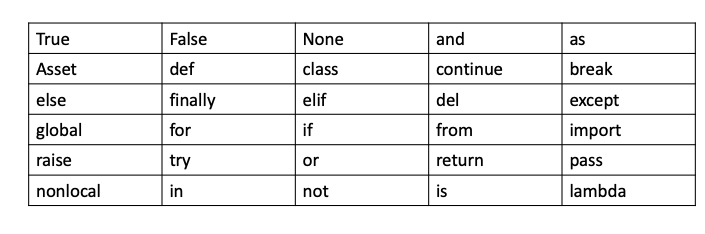
Identifiers
It is used to identify a variable, function, class, or object.
Rules for naming an identifier
- Keywords should not be used as an identifier.
- No special characters allowed except underscore( _ ) can be used as an identifier
- The first character of an identifier can be an alphabet or underscore( _ ) but cannot be a digit.
Literals
It is used to define the data as the variable
There are 4 types of literals are there
- String literals
- Numeric literals
- Boolean literals
- Special literals
Operators
Operators are the special symbols that are used to perform arithmetic and logical operations.
There are various types of operators are there:
- Arithmetic operators: It is used to perform common mathematical operations like Addition, Subtraction, Multiplication, Division, Modulus, and Exponentiations.
print('Addition:', 3 + 2)
# Addition: 5print('Subtraction:', 3 - 2)
#Subtraction: 1print('Multiplication:', 3 * 2)
# Multiplication: 6print('Division:', 3 / 2)
# Division: 1.5print('Exponentiation:', 9 ** 2)
# Exponentiation: 81print('Modulus:', 3 % 2)
# Modulus: 1
2.Assignment operators: It is used to assign values to the variables. Some of the common assignment operators are
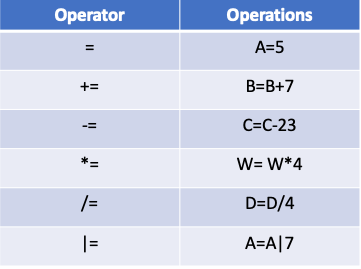
3.Comparison operators: It is used to compare values and return either True or False.
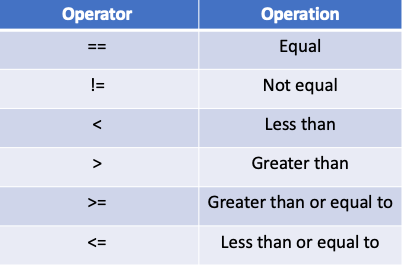
4.Logical operators: It is used to combine conditional statements.
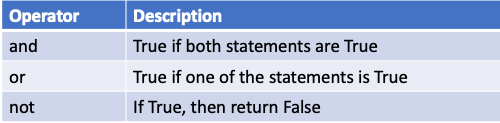
5.Bitwise operators: Used to compare binary numbers(0 or 1).
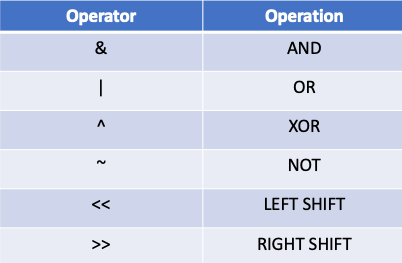
6.Identity operators: It is used to check if the objects are the same or not.
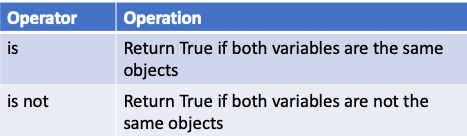
7.Membership operators: It is used to test if the sequence is present in an object.
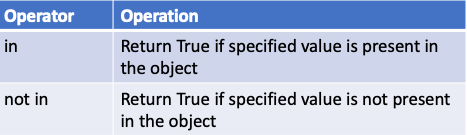
Conditional Statements
Conditions are the main features of any programming language. They perform different computations based on different conditions.
There are three types of conditional statements
The if
statement
In this conditional statements, if statements only execute if the given condition returns True.
The general syntax of an if the statement is:
if expression:
statement
The example is given below
if is_hot= True:
print('It is a hotter day')
The if-else statement
The statements inside the body of “if” only execute if the given condition returns true else it will execute the “else” part.
The general syntax of an if-else statement is:
if expression:
statement
else:
statement
The example is given below
if is_hot= True:
print('It is a hotter day')
else:
print('Beautiful day')
The elif
statements
We use elif is to provide a new condition to be checked before the else statement is executed.
if is_hot= True:
print('It is a hotter day')elif is_cold:
print('It is cold day')else:
print('Beautiful day')
Data types in Python
There are 4 data types in python
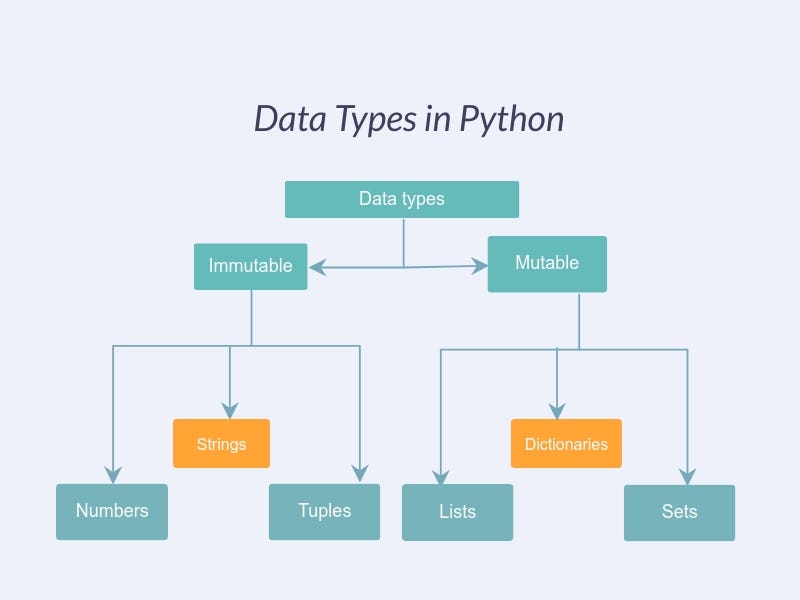
Strings
- To define strings we can use a single (‘ ‘) quotes or double (“ “) quotes.
To get a particular character in a string we use square brackets [ ].
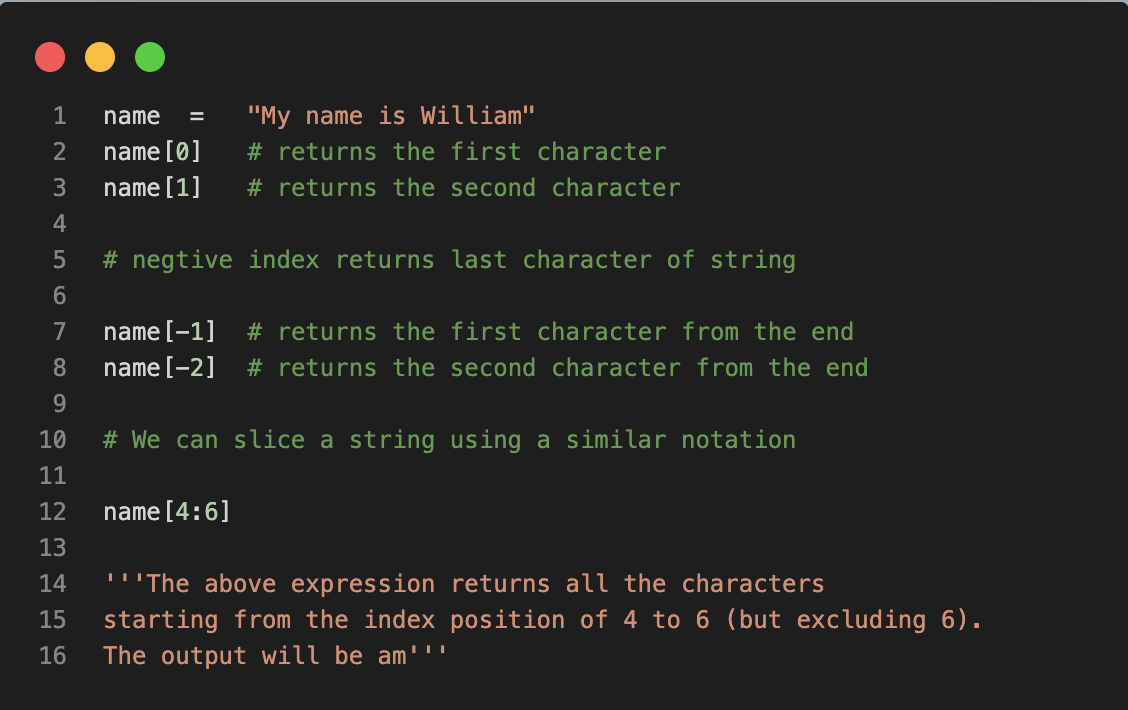
- To dynamically insert values into our strings we can use formatted strings.
name = ‘TOM’
message = f ’Hi, my name is {name}’Output: Hi, my name is TOM
Some common string methods are
- The upper() method returns the string in the upper case similarly the lower() method returns the string in the lower case.
- The title() method converts the first character of each word to upper case.
- The find() method searches the string for a specified value and returns the position of where it was found else print -1.
- The replace() method returns a string where a specified value is replaced with a specified value.
- in operator is used for checking if strings contain a character.
- capitalize() — -Converts the first character to upper case.
message = "Hi, My name is Sam"message.upper()
# 'HI, MY NAME IS SAM'message.lower()
# 'hi, my name is sam'message.title()
# 'Hi, My Name Is Sam'message.find(‘Sam’)
# 15message.find('b')
# -1message.replace(‘S’,’W’)
# 'Hi, My name is Wam'contains= ‘Abhi’ in messagemessage.capitalize()
# 'Hi, my name is sam'
Lists
A list is a data structure in which items are arranged in an order and have a definite count.
In Python, lists are written with square brackets denoted by [ ].
Some common list operations are
- To add items in a list we use the append() function. It will add the element in the last position.
thislist = [“apple”, “banana”, “cherry”]
thislist.append(“orange”)
print(thislist)
- To add items at a particular index we use insert() function.
thislist = ["apple", "banana", "cherry"]
thislist.insert(1, "orange")
print(thislist)# ['apple', 'orange', 'banana', 'cherry']
- To update the list we specify the position of the item to update and set the new value.
thislist = ["apple", "banana", "cherry"]
thislist[1]="Mango"
print(thislist)# ['apple', 'Mango', 'cherry']
- To remove items in a list we use remove() function
thislist = ["apple", "banana", "cherry"]
thislist.remove("banana")
print(thislist)# ['apple', 'cherry']
- To delete everything, use the
clear()
method
thislist = ["apple", "banana", "cherry"]
thislist.clear()
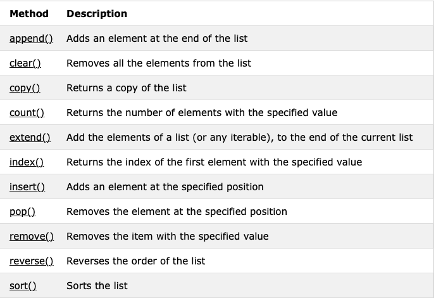
Tuples
- It is a collection of objects which is ordered and immutable.
- But once we define a tuple, we cannot add or remove items or change the existing item.
- In Python, tuples are denoted with round brackets ( ).
Lets us see one by one
Adding in a Tuple
Tuples are immutable. It means if we try to add a new item you will see an error.
thislist = ["apple", "banana", "cherry"]
thislist[3]= "Mango"
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: 'tuple' object does not support item assignment
Updating in a Tuple
If we try to update any item in tuple it will give us an error.
But there is a shortcut: you can convert into a list, change the item, and then convert it back to a tuple.
fruits = ["apple", "banana", "cherry"]
fruits_list = list(fruits)
fruits_list[1] = 'Mango'
fruits = tuple(fruits_list)
print(fruits)# ('apple', 'Mango', 'cherry')
Concatenating two or more tuples
We can concatenate two or more tuples by using + operator.
tuple1 = (“a”, “b” , “c”)
tuple2 = (1, 2, 3)tuple3 = tuple1 + tuple2
print(tuple3)# ('a', 'b', 'c', 1, 2, 3)
Deletion in a Tuple
Due to the immutable property, we can’t delete ab element in a tuple.
Retrieving an item in a Tuple
By using index method we can retrieve an item in tuple.
tuple1 = (“a”, “b” , “c”)
print(tuple1[1])# b
Checking for an existing item in a tuple
We can check for an existing item is present in a tuple or not by using anoperator.
fruits = ["apple", "banana", "cherry"]
if 'apple' in fruits:
print('We have apple')
else:
print('There is no apple')
Sets
A set is a data structure in which items are not in order so their index position also changes. In Python, sets are denoted with curly brackets.
Creating a Set
thisset = {“car”, “truck”, “bike”}
print(thisset)# {'car', 'bike', 'truck'}
Adding in a Set
To add an item in set we use add() method.
thisset.add('aeroplane')
Use the update()
method to add multiple items at once.
thisset.update(['skatebaord', 'bicycle'])
In sets repetition is not allowed. If we try to add multiple elements of same name it will count only one.
thisset = {“car”, “truck”, “bike”}people.add('truck')print(people)# {'car', 'bike', 'truck'}
Updating in a Set
Items in a set are not mutable. You have to either insert an item or delete an item.
Deleting in a Set
To remove Bob from the dictionary:
thisset = {“car”, “truck”, “bike”}
thisset.remove('car')
print(people)# {'bike', 'truck'}
To delete everybody:
thisset.clear()
Check if a given item already exists in a set
We use in operator to check if a given item is present in set or not.
thisset = {“car”, “truck”, “bike”}if 'car' in thisset:
print('car exists!')
else:
print('There is no car')
Dictionaries
- We use dictionaries to store key/value pairs.
- Dictionaries are mutable.
- In Python, dictionaries are denoted with curly brackets { }.
Adding in a Dictionary
If the key doesn’t exist yet, it is appended to the dictionary.
customer = {“name”: “John”, “age”: 24 }customer['Gender']="Male"
Updating a Dictionary
Let us update the existing value
#John's age is 42 now
customer['age']=42
Deleting in a Dictionary
customer.pop('age')
To delete everything:
customer.clear()
Retrieving in a Dictionary
john_age = customer['age']
print(john_age)24
Check if a given key already exists in a Dictionary
if 'age' in customer:
print('Age column exists')
else:
print('There is no age column')
Typecasting
It allows you to convert between different types. For example, we can convert int into str or a float to int.
There are two types of conversions are there
- Implicit conversion: In this type of conversion data type is automatically converted by the Python interpreter.
The example is shown given below
int_no = 24
float_no = 8.2
result = int_no + float_no
print(result)
# 35.2print(type(result))
# <class 'float'>
2. Explicit conversion: In this type of conversion users convert the data type of an object to the required data type.
The example is shown given below
string_no = str('23')
print(string_no)
#23# int to str
string_no = str(42)
print(string_no)
#42
# float to str
string_no = str(12.0)
print(string_no)
#12.0
Receiving Input
We can receive inputs from the user by calling the input() function.
name= input(‘what is your name’)
Note that the input() function always returns data as a string. So if we want to take input in integer, we have to convert it in integer.
age= int(input(‘what is your age’))
Looping Statements
It is a process in which we have a list of statements that executes repeatedly until it satisfies a condition.
There are 4 types of looping statements are there:
For loop
- It is used for repetition over a course(that is either a list, a tuple, a dictionary, a set, or a string).
The general syntax of for loops are:
for item in collection:
statement
The examples are given below
vehicles = [“car”, “bike”, “trucks”]
for i in vehicles:
print(i)
while loop
- This loop executes a set of statements as long as a condition is true.
The general syntax of while loops are
while condition:
statement
The examples are given below
i = 1
while i < 6:
print(i)
i += 1
break
- We use break statement to stop the iteration even if the while condition is true:
The examples are given below
i = 1
while i < 6:
print(i)
if i == 3:
break
i += 1
Continue
- continue statement is used to skip any current iteration and continue with the next.
The examples are given below
i = 1
while i < 6:
print(i)
if i == 3:
continue
i += 1
Functions on Python
- A function is a piece of code that is used to accomplish certain operations.
- def keyword is used for defining a function.
Syntax for defining a function is:
def function_name():
statements# To call a function
# use the function name followed by parenthesis:function_name()
Write a main function to print your name
def main():
print(“Hi, my name is Robin”)
return
a value
It is used to return a value from the function, it can either be a string or numeric or boolean.
The examples that are given below returns a string functions
def naming():
return 'sam'
print(naming())
By using return functions we can return multiple values.
def int_variable():
return 10,25
print(int_variable())# 10,25
Specifying the Arguments in the function definition
We can also pass arguments in given parenthesis
def function_name(person_name):
statementsfunction_name(“Watson”)
- We can pass multiple arguments inside the parenthesis
def add(a,b):
return a+badd(3,4)
- If the number of arguments is unknown, add a * before the parameter name:
def my_function(*kids):
print("The youngest child is " + kids[2])
my_function("Jenny", "Marry", "Damon")
Scope
A scope is a place where variables are created defines its availability to be accessed.
Global Scope:
In the global scope, variables can be accessed from anywhere in the program.
For example-
The code is given below
gender='male'
def print_gender():
print(' I am a ' + name + 'person.')
print_gender()#I am a male person
As you can see that we are accessing the gender variable from anywhere in the program.
Local Scope:
In local scope, when we declare a variable inside a function, it only exists inside that function and can’t be accessed from the outside.
For example- Suppose that you are a member of an environmental community. They have assigned a unique batch for you. Your batch is only known to community members. Not from anyone outside of the community.
def print_status():
status = "visible"
print(staus)
print_status()#visible
The variable status was declared inside the function.
But this will throw an error:
def print_status():
status = "visible"
print(staus)
print(status)
The output of the code above is:
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
NameError: name 'status' is not defined
When I tried to print the variable status from outside the function, but the scope of the variable was local and could not be found in a global scope.
To resolve this, we have to use global scope and local scope both to run the program.
status = "visible"def print_status():
status = "visible"
print(staus)print(print_status())
print(status)
Lambda Functions
Lambda expression is just like a shorter version of a function.
Syntax: lambda arguments : expression
g = lambda x: x*x*x
def cube(y):
return y*y*y;#instead of cube function we can write lambda functions
g = lambda x: x*x*x
Classes and Objects
Classes and Objects are the main concepts of Object-Oriented Programming.
- A Class is like a “blueprint” that defines variables and methods common to all objects.

- An Object is a collection of data and methods that acts on those data.
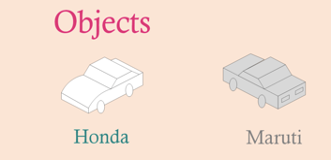
To define a class we use class keywords and after that, we create that we use that class name to create objects.
- Every class has an __init__() function, which is executed when the class is being initiated. And it is used to assign values to object properties that are necessary to do when the object is being created.
Write a program to create a class
- Create a class named Employee, use the __init__() function to assign values for employee_name, employee_id, and salary.
class Employee:
def __init__(self,employee_id, salary):
self.employee_name = employee_name
self.employee_id = employee_id
self.salary = salary def salary_increment(self):
self.salary += 5000
Let us explain the above block of code line by line.
- The class keyword is used to specify the name of the class Employee.
- Now we are using the __init__ method. It is used to an object that iscreated from a class and it allows the class to initialize the attributes of the class.
- The first parameter of __init__ function is self that is used to reference the object itself. Now we are creating another function that is used to increment the salary.
- Employee class has 3 attributes like employee_name, employee_id, and salary.
- Now we instantiate each object inside the __init__ method.
Now we create the objects . The code is down below.
p1= Employee("Sam",38,50000)print(p1.employee_name)
# Samprint(p1.employee_id)
# 38print(p1.salary)
# 50000p1.salary_increment()
print(p1.salary)
# 55000
Inheritance
- Inheritance is a technique to remove code duplication. We can create a parent class to define the common methods and then have child classes inherit these methods.
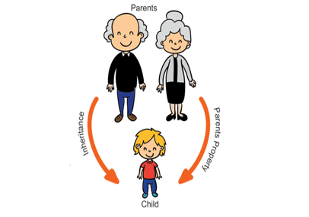
Let’s create a supercar class inherited from the car class.
class car:
def speed(self):
print("speed")class Supercar:
def price(self):
print("price")supercar= Supercar()
supercar.speed() # inherited from car
supercar.price() # defined in Supercar
Let’s break line by line that we have done:
- We have created a parent class which is a car and a child class which is Supercar.
- Now we have defined a speed method in the car class.
- Next, we have defined a price method in the Supercar class.
- Next, we created an object of the Supercar class.
- And called the speed method which the supercar has inherited from car class and price method.
Abstraction
- Abstraction is a mechanism that lets you think about the big picture without worrying about the details.
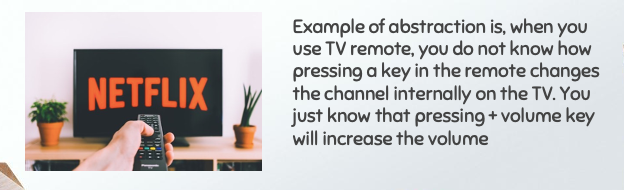
Modules
- A module can have a set of functions, classes, or variables defined and implemented.
- To import a module we use the import keyword.
>>> import math>>>math.pi
3.141592653589793>>>math.radians(30)
0.5235987755982988# To calculate power of a no
>>>math.pow(2,4)
16.0# To calculate square root>>>math.sqrt(100)
10.0# ceil gives the nearest integer to a value
>>>math.ceil(4.5867)
5
Regular Expressions
- It is used to match character combinations in strings.
- RegEx can be utilized to check if a string contains the predefined search pattern.
- It is often using cleaning text data in natural language processing.
Some Common functions in a regex are
findall() Function
- It is used to return a list containing all matches.
import retxt = ”Data Spoof is a place to learn artificial intelligence.”
x = re.findall(”oo”, txt)
print(x)
search() Function
- This function searches the string for a match and returns a Match object if there is a match.
- If there is more than one match, just the first occurrence of the match will be returned.
import retxt = ”Data Spoof is a place to learn”
x = re.search(“\s”, txt)print(“The first white-space character is located in position:”, x.start())
split( ) Function
- This function is used to returns a list where the string has been split at each match.
import retxt = “The rain in France”
x = re.split(“\s”, txt)
print(x)
sub( ) Function
- The sub( ) function replaces the matches with the text of your choice.
import retxt = ”Data Spoof is a place to learn”
x = re.sub(“\s”, “9”, txt)
print(x)
File Handling
- It has a mechanism to store the results of a program in a file and perform various operations in it.
- It has many python functions for creating, reading, updating, and deleting files.
- To open files in Python we use the open() function. It takes two arguments; filename, and mode.
There are 4 different types of modes
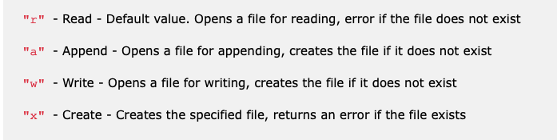
Syntax in python is given below
f = open(”file_name.txt”,”modes”)
How to read a file in python
- First, we have to open a file and then read it using the read function.
f = open("demofile.txt", "r")
print(f.read())# For reading a single line we use readline() function.
print(f.readline())# For reading only few characters we use
print(f.read(5))
It is always good to close the file after using by using close () function.
How to use write method in python
- To keep in touch with a current file, you must add a parameter to the open() function:
- We use “a”(append) function to add the text to the end of the file.
f = open("demofile2.txt", "a")
f.write("Now the file has more content!")
f.close()
- ”w” — Write — will overwrite any existing content
f = open("demofile3.txt", "w")
f.write("OOP's! I have deleted the content!")
f.close()
How to delete a file
- To delete or remove a file we use os.remove() function.
import osif os.path.exists("demofile.txt"):
os.remove("demofile.txt")
else:
print("The file does not exist")
Exception Handling
- When an error occurs, or exception as we call it, Python will typically stop and create an error message.
- To handle those exceptions we use to try and except block.
x=5/0 try:
print(x)
except:
print(“An exception occurred”)# Dividing by zero!
- The finally block will be executed anyhow if the try block raises an error or not.
try:
print(x)
except:
print(“Something went wrong”)
finally:
print(“The ‘try except’ is finished”)
- You can choose to throw a custom exception if an error condition occurs.
- To throw an exception, use the raise keyword.
x = -1if x < 0:
raise Exception(“Sorry, no numbers below zero”)
Conclusion
Finally, we made it to the end of the tutorial. We have learned all the basic concepts of python and it’s implementation.